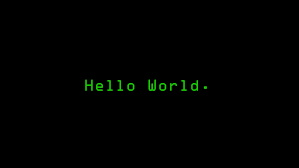
I guess I wrote my R “hello world!” function 7 or 8 years ago while approaching R for the first time. And it is too little to illustrate the basic syntax of a programming language for a working program to a wannabe R programmer. Thus, here follows a collection of basic functions that may help a bit more than the famed piece of code.
###################################################### ############### Hello world functions ################ ###################################################### ################################## # General info fun <- function( arguments ) { body } ################################## foo.add <- function(x,y){ x+y } foo.add(7, 5) ---------------------------------- foo.above <- function(x){ x[x>10] } foo.above(1:100) ---------------------------------- foo.above_n <- function(x,n){ x[x>n] } foo.above_n(1:20, 12) ---------------------------------- foo = seq(1, 100, by=2) foo.squared = NULL for (i in 1:50 ) { foo.squared[i] = foo[i]^2 } foo.squared ---------------------------------- a <- c(1,6,7,8,8,9,2) s <- 0 for (i in 1:length(a)){ s <- s + a[[i]] } s ---------------------------------- a <- c(1,6,7,8,8,9,2,100) s <- 0 i <- 1 while (i <= length(a)){ s <- s + a[[i]] i <- i+1 } s ---------------------------------- FunSum <- function(a){ s <- 0 i <- 1 while (i <= length(a)){ s <- s + a[[i]] i <- i+1 } print(s) } FunSum(a) ----------------------------------- SumInt <- function(n){ s <- 0 for (i in 1:n){ s <- s + i } print(s) } SumInt(14) ----------------------------------- # find the maximum # right to left assignment x <- c(3, 9, 7, 2) # trick: it is necessary to use a temporary variable to allow the comparison by pairs of # each number of the sequence, i.e. the process of comparison is incremental: each time # a bigger number compared to the previous in the sequence is found, it is assigned as the # temporary maximum # Since the process has to start somewhere, the first (temporary) maximum is assigned to be # the first number of the sequence max <- x[1] for(i in x){ tmpmax = i if(tmpmax > max){ max = tmpmax } } max x <- c(-20, -14, 6, 2) x <- c(-2, -24, -14, -7) min <- x[1] for(i in x){ tmpmin = i if(tmpmin < min){ min = tmpmin } } min ---------------------------------- # n is the nth Fibonacci number # temp is the temporary variable Fibonacci <- function(n){ a <- 0 b <- 1 for(i in 1:n){ temp <- b b <- a a <- a + temp } return(a) } Fibonacci(13) ---------------------------------- # R available factorial function factorial(5) # recursive function: ff ff <- function(x) { if(x<=0) { return(1) } else { return(x*ff(x-1)) # function uses the fact it knows its own name to call itself } } ff(5) ---------------------------------- say_hello_to <- function(name){ paste("Hello", name) } say_hello_to("Roberto") ---------------------------------- foo.colmean <- function(y){ nc <- ncol(y) means <- numeric(nc) for(i in 1:nc){ means[i] <- mean(y[,i]) } means } foo.colmean(airquality) ---------------------------------- foo.colmean <- function(y, removeNA=FALSE){ nc <- ncol(y) means <- numeric(nc) for(i in 1:nc){ means[i] <- mean(y[,i], na.rm=removeNA) } means } foo.colmean(airquality, TRUE) ---------------------------------- foo.contingency <- function(x,y){ nc <- ncol(x) out <- list() for (i in 1:nc){ out[[i]] <- table(y, x[,i]) } names(out) <- names(x) out } set.seed(123) v1 <- sample(c(rep("a", 5), rep("b", 15), rep("c", 20))) v2 <- sample(c(rep("d", 15), rep("e", 20), rep("f", 5))) v3 <- sample(c(rep("g", 10), rep("h", 10), rep("k", 20))) data <- data.frame(v1, v2, v3) foo.contingency(data,v3)That's all folks! #R #rstats #maRche #Rbloggers This post is also shared in LinkedIn and www.r-bloggers.com
I think something went wrong the formatting of the R code (lots of HTML tags included).
Thanks Holger, it is now fixed.
forgot a “with” 😉